php isn’t the sort of language where developers usually think about things like memory. we just sort of sling around variables and functions and let the internals figure out all that ‘ram stuff’ for us. let’s change that.
in first part of this series, we built a php script that was able to run a number of tasks concurrently by forking child processes. it worked pretty well, but there was a glaring, unaddressed problem: there was no way for those child processes to send data back to the parent process.
in this installment, we’re going to solve that issue by using shmop
, php’s “shared memory operations”.
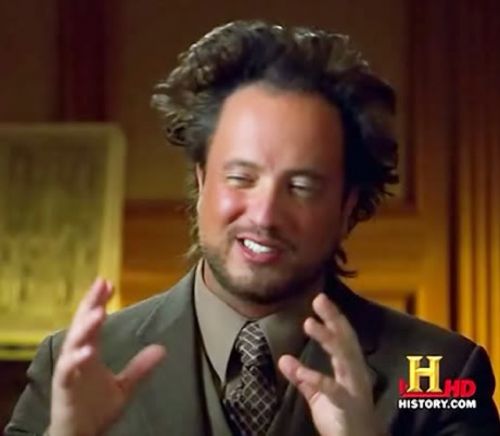